2 years ago
#46110
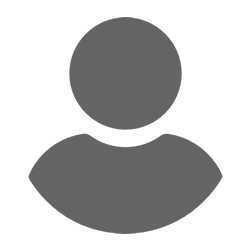
user17056867
Flask socket with Pool
I am trying to implement the following:
from multiprocessing import Pool
from flask import Flask, jsonify
from flask_cors import CORS
from flask_socketio import SocketIO, send, emit
app = Flask(__name__)
CORS(app)
socketio = SocketIO(app, cors_allowed_origins="*")
@socketio.on('connect')
def connect():
emit('result', {'data': 'Connected'})
def ab_test(data):
socketio.emit('result', {'data': data})
return "OK"
@app.route('/api/test', methods=['POST'])
def test():
users = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]
with Pool(2) as p:
data_pool = users
result = p.map(ab_test, data_pool)
return jsonify({"status": "complete"})
if __name__ == '__main__':
socketio.run(app, debug=True)
Please explain to me why when using Pool my messages are not sent to the Client via socket?
For example , if you do this , then everything works:
def ab_test(data):
socketio.emit('result', {'data': data})
return "OK"
@app.route('/api/test', methods=['POST'])
def test():
users = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]
for user in users:
ab_test(user)
return jsonify({"status": "complete"})
python
sockets
flask-socketio
0 Answers
Your Answer